
Using Docker Buildx and Docker Bake to Build Images
Feb 19
4 min read
0
3
In the world of software development and DevOps, optimizing the process of building, testing, and deploying applications is crucial. Docker has become a dominant technology for containerization, providing tools that facilitate the easy creation and management of container images. Among its features, Docker Buildx and Docker Bake stand out as powerful tools for building images. In this blog post, we will explore these tools, their benefits, and how to effectively use them in your workflows.
Understanding Docker Buildx
Docker Buildx is an extension of the Docker CLI. It allows you to create, manage, and dispatch builds to various output destinations. Buildx supports multi-platform builds and seamlessly integrates with Docker's BuildKit features.
One of the key advantages of Buildx is its ability to build images targeting multiple platforms. For instance, if you want to create images for both ARM and x86 architectures, you can do so without needing to run separate build commands.
Why Use Buildx?
Multi-Platform Support: Buildx allows you to specify multiple target platforms in a single command, reducing complexity.
Enhanced Performance: By leveraging BuildKit, Buildx is optimized for faster builds through parallelism and caching.
Improved Customization: Advanced features such as build contexts and custom caches make it easier to tweak builds according to your project’s requirements.
To start using Buildx, you need to enable BuildKit first. You can do this by setting an environment variable in your terminal:
```bash
export DOCKER_BUILDKIT=1
```
Now, you can create a new builder instance using the following command:
```bash
docker buildx create --name mybuilder
docker buildx use mybuilder
```
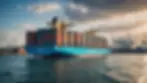
This command will create a new Buildx instance called `mybuilder`. Once created, you can run your build with:
```bash
docker buildx build --platform linux/arm64,linux/amd64 -t my-image:latest .
```
This command builds your image for both ARM and AMD architectures, and tags it as `my-image:latest`.
Introduction to Docker Bake
Docker Bake is another powerful tool designed to streamline the image building process, operating in conjunction with Docker Buildx. It enables you to define and manage complex builds using a simple configuration file known as a `docker-bake.hcl` or a corresponding JSON file.
Benefits of Using Docker Bake
Simplicity in Configuration: With Docker Bake, complex build configurations can be declared in one central file, making them easier to manage.
Parallel Builds: Bake can execute multiple build processes concurrently, significantly reducing the time taken to compile images.
Reusable Build Specifications: You can define reusable build specifications, making it easier to maintain consistency across multiple projects.
To get started with Docker Bake, create a `docker-bake.hcl` file in your project directory:
```hcl
group "default" {
targets = ["frontend", "backend"]
}
target "frontend" {
context = "./frontend"
dockerfile = "Dockerfile"
tags = ["my-image/frontend:latest"]
}
target "backend" {
context = "./backend"
dockerfile = "Dockerfile"
tags = ["my-image/backend:latest"]
}
```
Using this configuration, you can build your images by simply executing the following command:
```bash
docker buildx bake
```
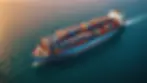
This command will look for the `docker-bake.hcl` file in the current directory and build both the frontend and backend images simultaneously.
Advanced Use Cases for Docker Buildx and Bake
Writing Custom Build Scripts
Sometimes, you might have unique requirements that necessitate custom build scripts. Buildx supports custom build flags, which allow you to tailor the build process even further. For example, you can use build arguments to dynamically set the environment during the build.
Here is how you can utilize build arguments in your `Dockerfile`:
```Dockerfile
ARG VERSION=latest
FROM my-image:${VERSION}
```
You can then set the build argument when calling Buildx:
```bash
docker buildx build --build-arg VERSION=1.0 -t my-image:1.0 .
```
Integrating with CI/CD Pipelines
Docker Buildx and Bake can be easily integrated into your Continuous Integration/Continuous Deployment (CI/CD) workflows. Many CI/CD tools, like Jenkins or GitHub Actions, allow you to run shell commands as part of the build process.
For instance, if you're using GitHub Actions, you can add a step to your workflow configuration that uses Docker Buildx to build your images:
```yaml
name: Build and Push
on:
push:
branches:
- main
jobs:
build:
runs-on: ubuntu-latest
steps:
- name: Checkout code
uses: actions/checkout@v2
- name: Set up Docker Buildx
uses: docker/setup-buildx-action@v1
- name: Build and push images
run: |
echo "${{ secrets.DOCKER_USERNAME }}" | docker login -u "${{ secrets.DOCKER_USERNAME }}" --password-stdin
docker buildx bake
```
This YAML snippet showcases the integration of Docker Bake into a CI/CD pipeline, allowing seamless image builds upon each push to the `main` branch.
Monitoring and Troubleshooting Builds
As with any development process, monitoring and troubleshooting are vital. Docker Buildx and Bake provide useful output information to help you diagnose issues quickly.
By default, Buildx outputs logs that indicate which steps are running and any errors encountered. You can increase logging verbosity for more detailed stories by adjusting the `--progress` flag:
```bash
docker buildx build --progress=plain .
```
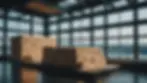
Using `--progress=plain` allows you to see step-by-step output, making it easier to spot where a build might be failing.
Final Thoughts on Docker Buildx and Bake
Docker Buildx and Bake have revolutionized the way developers and DevOps engineers create and manage container images. By allowing for multi-platform builds, simplified configurations, and integration into CI/CD pipelines, they enhance productivity and efficiency.
Understanding how to effectively use these tools can lead to smoother deployment processes and better resource utilization. By adopting Docker Buildx and Docker Bake in your workflows, you can stay ahead in the fast-paced world of containerized application development.
As you explore these tools further, be sure to refer to the official Docker documentation for the latest updates and advanced features. Happy building!